JSON Minifier: How to Compress JSON Files for Better Web Performance
In the era of web applications, JSON (JavaScript Object Notation) has become the standard format for data exchange. However, as the complexity and size of JSON files increase, the need for optimization becomes apparent. JSON Minifier is a tool that reduces the size of JSON files by removing unnecessary characters like whitespaces and line breaks, without altering the data. This article delves into the importance of JSON minification, how it works, and the best tools available to get the job done efficiently.
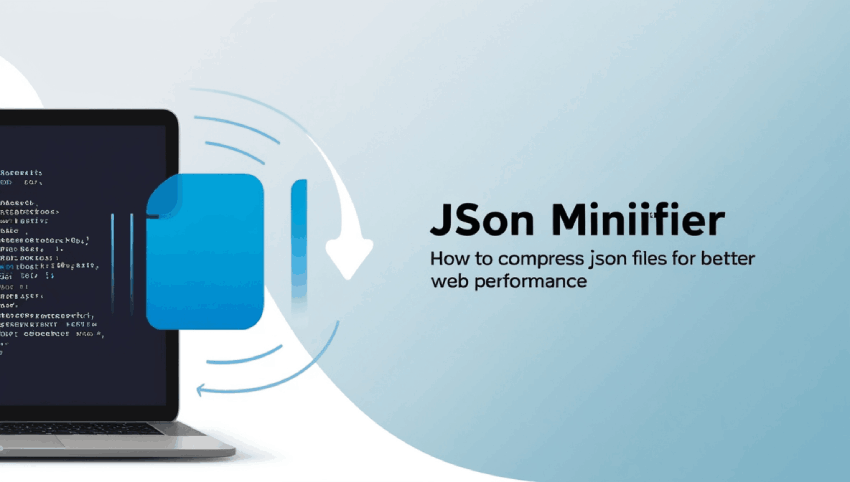
What is JSON Minifier?
A JSON Minifier is a tool used to compress JSON files by stripping out unnecessary characters such as whitespaces, comments, and newlines. While JSON is designed to be human-readable, these extra characters add to the file size, increasing download times. By using a minifier, you can reduce the file size, which in turn boosts web performance and decreases load times.
For example, here’s a sample JSON file:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"isStudent": false
}
After minification, the file looks like this:
{"name":"John Doe","age":30,"city":"New York","isStudent":false}
Although the content remains the same, the file size is now smaller and loads faster when fetched by a browser or server.
Why Should You Minify JSON Files?
Minifying JSON files offers several benefits, particularly for web development and API communication:
- Faster Data Transfer: Smaller files transfer more quickly across networks, especially on mobile networks or slower connections.
- Improved Web Performance: Reducing the size of JSON files can lead to faster page loads, improving the overall user experience.
- Reduced Server Load: Smaller JSON files reduce bandwidth usage, which is beneficial for websites or applications with high traffic.
- Better API Performance: Minified JSON files are useful in API responses, speeding up the communication between client and server.
How to Minify JSON Files
There are several methods to minify JSON files, from online tools to integrating minification in your development workflow. Let’s explore some of the most popular options.
Using Online JSON Minifier Tools
For quick and easy minification, online tools are a convenient option. Some of the best free JSON Minifier tools include:
- JSONLint - A validator and minifier tool that allows you to paste your JSON and get a minified version instantly.
- JSONFormatter - A user-friendly tool for both formatting and minifying JSON data.
- FreeFormatter - Another reliable tool for validating and minifying JSON files.
Simply copy and paste your JSON into these tools and click “Minify” to get a compact version of the file.
Minifying JSON with JavaScript
If you working on a JavaScript project and want to incorporate JSON minification directly into your code, the JSON.stringify
method in JavaScript can help. Here’s a simple example:
let jsonObject = {
"name": "John Doe",
"age": 30,
"city": "New York"
};
let minifiedJSON = JSON.stringify(jsonObject);
console.log(minifiedJSON); // Outputs: {"name":"John Doe","age":30,"city":"New York"}
This code converts a JSON object into a minified string without any extra characters. You can further integrate this into your application workflow or backend systems to ensure JSON files are always minified before being served to clients.
Minifying JSON in Build Tools
For developers working on large projects, automating the minification process with build tools is recommended. Some of the most popular build tools for this include:
- Gulp: With the
gulp-jsonminify
plugin, you can automate JSON minification during your build process. - Grunt: The
grunt-json-minify
plugin is another useful tool to integrate JSON minification into your Grunt tasks. - Webpack: Webpack allows you to manage and optimize your assets, and with proper configurations, you can minify JSON files automatically.
By integrating JSON minification into your build process, you can ensure that every time you deploy your project, all JSON files are optimized for size and performance.
Common Mistakes When Minifying JSON
Although JSON minification is a straightforward process, there are a few common mistakes developers should avoid:
- Removing Necessary Characters: Some minification tools or manual processes might inadvertently remove essential characters or syntax, leading to errors.
- Over-Minifying: Compressing JSON too aggressively can make it difficult to debug or work with the file during development.
- Skipping Validation: Always validate your JSON before and after minification to ensure that the file is still properly structured and error-free.
Best Practices for JSON Minification
Here are some best practices to follow when minifying JSON files:
- Use Reliable Tools: Always use trusted tools or libraries for JSON minification to avoid introducing errors or losing important data.
- Validate Before and After: Validate your JSON both before and after minification to ensure data integrity and avoid any issues.
- Test on All Browsers: Make sure the minified JSON works correctly across all major browsers and devices to ensure compatibility.
- Automate the Process: Incorporate JSON minification into your build or deployment pipeline using tools like Gulp or Webpack.
Conclusion
Using a JSON Minifier is a simple yet effective way to reduce the size of JSON files, enhancing your web application's performance. Whether you're a developer working on small projects or managing large-scale applications, minifying your JSON files will reduce load times, improve user experience, and optimize data transfer. By following the best practices and avoiding common mistakes, you can ensure that your JSON files are always optimized for performance.
JSON Syntax
JSON data is written as key/value pairs, similar to JavaScript objects. Here the basic syntax:
{
"name": "John",
"age": 30,
"city": "New York"
}
In this example, `name`, `age`, and `city` are keys, and "John", 30, and "New York" are their corresponding values.
JSON Data Types
JSON supports the following data types:
- String: A sequence of characters enclosed in double quotes. Example: `"Hello"`
- Number: A numerical value. Example: `25`
- Boolean: Represents `true` or `false`. Example: `true`
- Array: An ordered list of values enclosed in square brackets. Example: `["apple", "banana", "cherry"]`
- Object: An unordered collection of key/value pairs enclosed in curly braces. Example: `{"name": "John", "age": 30}`
- Null: Represents an empty value. Example: `null`
JSON Example
Here is an example of a more complex JSON object that contains an array and nested objects:
{
"name": "Alice",
"age": 25,
"address": {
"street": "123 Main St",
"city": "Wonderland"
},
"hobbies": ["reading", "chess", "hiking"]
}
In this example, the `address` is a nested object, and `hobbies` is an array of strings.
How to Use JSON
JSON is most commonly used for transferring data between a client and a server. Here’s an example of how JSON might be used in JavaScript:
Parsing JSON
You can convert a JSON string into a JavaScript object using the JSON.parse()
method:
let jsonString = '{"name": "Bob", "age": 22}';
let obj = JSON.parse(jsonString);
console.log(obj.name); // Output: Bob
Stringifying JSON
You can convert a JavaScript object into a JSON string using the JSON.stringify()
method:
let obj = { name: "Bob", age: 22 };
let jsonString = JSON.stringify(obj);
console.log(jsonString); // Output: {"name":"Bob","age":22}
Benefits of JSON
JSON is widely used because:
- It is lightweight and easy to read.
- It is language-independent, meaning it can be used with any programming language.
- It integrates well with most APIs and web services.
- It allows for easy transmission of complex data structures like arrays and nested objects.
Conclusion
JSON is a powerful tool for data exchange between client and server in modern web development. Understanding its syntax and how to manipulate it with JavaScript is essential for working with APIs and dynamic web applications.