JavaScript Minification: A Complete Guide to Boosting Web Performance
In today’s competitive digital landscape, website performance is crucial for providing an optimal user experience. One key factor affecting website speed is the size of the files served to users, particularly JavaScript files. JavaScript Minification is an effective technique to reduce file sizes, thereby improving load times and overall site performance. This article provides an in-depth guide to JavaScript Minification, covering its importance, methods, common pitfalls, and best practices.
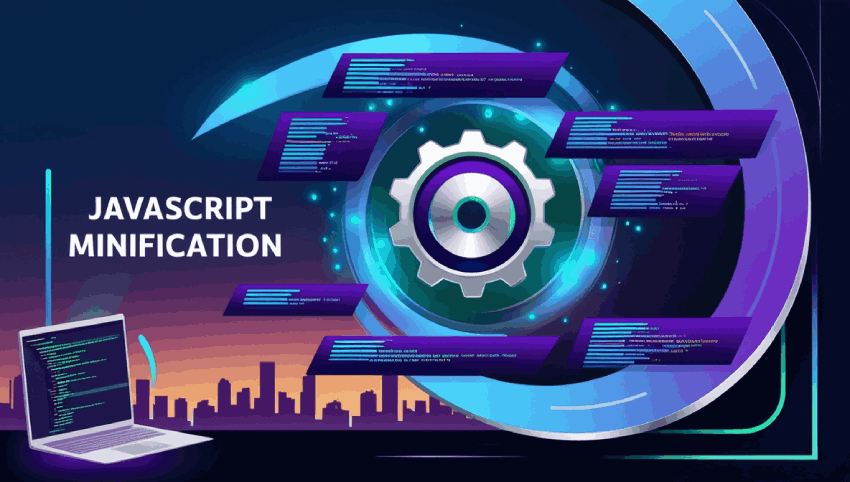
What is JavaScript Minification?
JavaScript Minification is the process of compressing JavaScript code by removing all unnecessary characters, such as whitespaces, comments, and line breaks, without changing its functionality. The goal is to reduce the size of the JavaScript file to decrease download times and improve website speed.
For example, consider the following JavaScript code:
// This is a sample JavaScript function
function greetUser(name) {
console.log("Hello, " + name);
}
greetUser("John");
After minification, the code looks like this:
function greetUser(name){console.log("Hello, "+name)}greetUser("John");
By eliminating unnecessary characters, the file size is significantly reduced, leading to faster loading times, especially for sites that rely heavily on JavaScript.
Why is JavaScript Minification Important?
Minifying JavaScript files offers several benefits:
- Improved Loading Speed: Reducing the size of JavaScript files allows browsers to load them faster, resulting in quicker page rendering.
- Reduced Bandwidth Usage: Smaller files consume less bandwidth, which is particularly important for users on mobile networks or sites with high traffic.
- Better User Experience: Faster websites provide a smoother user experience, leading to lower bounce rates and higher engagement.
- SEO Benefits: Search engines like Google prioritize fast-loading websites, so minification can help boost your site’s search rankings.
How to Minify JavaScript
There are several ways to minify JavaScript, ranging from online tools to build tools that automate the process. Below, we explore some of the most common methods.
Using Online Tools for JavaScript Minification
If you need a quick and easy solution to minify your JavaScript code, online tools are a great option. Here are a few popular JavaScript minification tools:
- JavaScript Minifier - A simple tool where you can paste your JavaScript code and instantly receive a minified version.
- MinifyJS - Provides additional options for compressing your JavaScript files while maintaining code integrity.
- UglifyJS - A popular and powerful tool for JavaScript minification that includes various customization options.
To use these tools, simply copy and paste your JavaScript code into the provided field, click the minify button, and download the minified version.
Minifying JavaScript with Build Tools
For larger projects, incorporating JavaScript minification into your build process is more efficient. Here are some popular build tools that support JavaScript minification:
- Webpack: A powerful module bundler for JavaScript that can minify JavaScript using the
TerserPlugin
during the build process. - Gulp: A task runner that allows you to automate minification using plugins like
gulp-uglify
orgulp-terser
. - Grunt: Another task runner that simplifies development tasks. With the
grunt-contrib-uglify
plugin, you can easily minify JavaScript files.
Using these tools helps streamline the minification process by automatically minifying JavaScript files every time the project is built.
Manual JavaScript Minification
If you prefer full control over the process, you can manually minify your JavaScript files. This method is generally not recommended for large projects as it can be time-consuming and prone to errors. However, for small files, you can follow these steps:
- Remove all comments and unnecessary whitespace.
- Shorten variable names where applicable.
- Eliminate redundant or unused code.
- Compress and concatenate multiple JavaScript files into one, if possible.
Manual minification is less efficient and more prone to human error, so it generally recommended to use automated tools whenever possible.
Common Mistakes in JavaScript Minification
While JavaScript Minification is a useful technique, there are several common mistakes that developers should avoid:
- Removing Necessary Whitespace: Unlike HTML, JavaScript often relies on whitespace for proper syntax. Removing too much whitespace can cause the code to break.
- Over-Minification: In an attempt to save more space, some developers may over-minify their code, which can make it difficult to debug and maintain.
- Not Testing Minified Files: After minifying your JavaScript files, always test them in various environments to ensure they work as expected.
- Using Unreliable Minifiers: Some minification tools may produce errors or introduce bugs. It important to use reputable tools or libraries to avoid issues.
Best Practices for JavaScript Minification
To ensure the success of your minification process, follow these best practices:
- Automate Minification: Use build tools like Webpack, Gulp, or Grunt to automate the minification process. This ensures that your JavaScript files are always minified before deployment.
- Test Minified Code: Always test the minified versions of your JavaScript files in various browsers and environments to ensure compatibility.
- Version Control: Keep both the original and minified versions of your JavaScript files in version control to easily track changes and revert if needed.
- Combine Files: Where possible, combine multiple JavaScript files into a single file to reduce HTTP requests and further improve performance.
- Use Source Maps: Enable source maps during development to make it easier to debug minified code.
Conclusion
JavaScript Minification is a powerful technique to enhance web performance by reducing the size of JavaScript files, leading to faster load times, improved SEO rankings, and better user experiences. Whether you use online tools, automated build tools, or manual methods, minifying your JavaScript code is an essential step toward optimizing your website performance.
Start Minifying Your JavaScript Today!
Take the first step in improving your website’s speed and performance by implementing JavaScript Minification. Use the tools and techniques outlined in this guide, and watch your site’s load times improve!
JavaScript Syntax
JavaScript syntax is the set of rules that define a correctly structured JavaScript program. Here is a simple example:
// This is a comment
let message = "Hello, world!";
console.log(message); // Output: Hello, world!
In this example, `let` is used to declare a variable, and `console.log()` is used to print a message to the browser's console.
JavaScript Variables
Variables in JavaScript are used to store data values. You can declare a variable using var
, let
, or const
. Here's how:
var x = 5;
let y = 10;
const z = 15;
console.log(x + y + z); // Output: 30
The main difference between var
, let
, and const
is their scope. const
is used to declare variables that won't change, while var
and let
can be reassigned.
JavaScript Data Types
JavaScript supports various data types such as:
- Number: e.g.,
42
- String: e.g.,
"Hello"
- Boolean: e.g.,
true
orfalse
- Array: e.g.,
[1, 2, 3]
- Object: e.g.,
{name: "Alice", age: 25}
JavaScript Functions
Functions are blocks of code designed to perform a particular task. They are executed when "called" or "invoked." Here's a simple function:
function greet(name) {
return "Hello, " + name;
}
console.log(greet("John")); // Output: Hello, John
The function `greet` takes a parameter `name` and returns a greeting string. You can call it multiple times with different arguments.
JavaScript Conditionals
Conditional statements control the flow of a program. The most common conditional is the `if` statement:
let age = 18;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
This code checks whether the variable `age` is greater than or equal to 18, and logs different messages based on the result.
JavaScript Loops
Loops allow you to repeat code a number of times. Here an example of a `for` loop that iterates over an array:
let fruits = ["apple", "banana", "cherry"];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
// Output: apple, banana, cherry
The loop iterates over the `fruits` array and prints each fruit to the console.
JavaScript Arrays
Arrays are used to store multiple values in a single variable. You can access elements in an array using their index:
let colors = ["red", "green", "blue"];
console.log(colors[0]); // Output: red
JavaScript arrays come with many built-in methods, such as push()
to add an element to the array, and pop()
to remove the last element.
JavaScript Objects
Objects are used to store keyed collections of data. Here an example:
let person = {
name: "Alice",
age: 30,
city: "Wonderland"
};
console.log(person.name); // Output: Alice
In this example, the object `person` has properties `name`, `age`, and `city`, which can be accessed using dot notation.
JavaScript Events
JavaScript can interact with HTML elements through events. For example, you can execute JavaScript code when a user clicks a button:
<button onclick="displayMessage()">Click Me!</button>
<script>
function displayMessage() {
alert("Hello, world!");
}
</script>
In this example, the `displayMessage` function will be called when the button is clicked, displaying an alert box with the message "Hello, world!"
Conclusion
JavaScript is an essential tool for web development. By mastering its syntax, variables, functions, conditionals, loops, arrays, objects, and event handling, you be able to create dynamic and interactive web applications.